Calculation method of gray value
三种不同计算灰度的方式
ShaderToy https://www.shadertoy.com/view/mlVBRh
Code:
/*
https://www.shadertoy.com/view/mlVBRh
Blog:https://blog.lovezjj.cn
by cfl997 20231207
*/
//Green
vec3 GrayByGreen(vec3 color)
{
return vec3(color.g);
}
//Gray = R*0.3 + G*0.59 + B*0.11
const vec3 floatcoefficient = vec3(0.3,0.59,0.11);
vec3 GrayByfloat(vec3 color)
{
float w=dot(color,floatcoefficient);
return vec3(w);
}
//Average
vec3 GrayByAverage(vec3 color)
{
float w=(color.r+color.g+color.b)/3.;
return vec3(w);
}
void mainImage( out vec4 fragColor, in vec2 fragCoord )
{
vec2 uv = fragCoord/iResolution.xy;
vec3 color=texture(iChannel0,uv).rgb;
uv*=vec2(3.,1.);
if(uv.x<0.95) {color=texture(iChannel0,uv).rgb; color=GrayByGreen(color);}
else if(uv.x>1.&&uv.x<1.95) {uv.x-=1.;color=texture(iChannel0,uv).rgb; color=GrayByfloat(color);}
else if(uv.x>2.&&uv.x<3.) {uv.x-=2.;color=texture(iChannel0,uv).rgb; color=GrayByAverage(color);}
else {color=vec3(1.0);}
fragColor=vec4(color,1.);
}
详解:
这段代码是一个用于在ShaderToy上显示的着色器程序,它主要实现了将输入纹理(iChannel0
)按照不同的灰度处理方式进行转换,并将结果显示在屏幕上。以下是对代码的详细解释:
//Green
vec3 GrayByGreen(vec3 color)
{
return vec3(color.g);
}
这个函数 GrayByGreen
接收一个颜色向量 color
,然后返回一个新的颜色向量,其中只保留了原始颜色向量中的绿色分量,而其他分量设为0。这实际上将原始颜色转换为灰度图像,只使用了绿色通道的信息。
//Gray = R*0.3 + G*0.59 + B*0.11
const vec3 floatcoefficient = vec3(0.3, 0.59, 0.11);
vec3 GrayByfloat(vec3 color)
{
float w = dot(color, floatcoefficient);
return vec3(w);
}
这个函数 GrayByfloat
使用了常量系数 floatcoefficient
,将输入颜色向量 color
转换为灰度图像。通过使用红、绿、蓝通道的不同权重,这里采用了一个标准的灰度转换公式。
//Average
vec3 GrayByAverage(vec3 color)
{
float w = (color.r + color.g + color.b) / 3.0;
return vec3(w);
}
这个函数 GrayByAverage
计算输入颜色向量 color
的平均值,从而将其转换为灰度图像。
void mainImage(out vec4 fragColor, in vec2 fragCoord)
{
vec2 uv = fragCoord / iResolution.xy;
vec3 color = texture(iChannel0, uv).rgb;
uv *= vec2(3.0, 1.0);
if (uv.x < 0.95)
{
color = texture(iChannel0, uv).rgb;
color = GrayByGreen(color);
}
else if (uv.x > 1.0 && uv.x < 1.95)
{
uv.x -= 1.0;
color = texture(iChannel0, uv).rgb;
color = GrayByfloat(color);
}
else if (uv.x > 2.0 && uv.x < 3.0)
{
uv.x -= 2.0;
color = texture(iChannel0, uv).rgb;
color = GrayByAverage(color);
}
else
{
color = vec3(1.0);
}
fragColor = vec4(color, 1.0);
}
最后,mainImage
函数是ShaderToy中的主函数,它通过对输入纹理进行不同的灰度处理,根据屏幕坐标 fragCoord
计算出相应的纹理坐标 uv
。然后,根据 uv.x
的值不同,分别调用不同的灰度处理函数,最终将结果赋给 fragColor
,用于显示在屏幕上。
The provided shader code is a program designed for ShaderToy, a platform for creating and sharing shader programs. The code implements various grayscale conversion methods on an input texture (iChannel0
) and displays the results on the screen. Here’s a detailed explanation of the code:
//Green
vec3 GrayByGreen(vec3 color)
{
return vec3(color.g);
}
The GrayByGreen
function takes a color vector color
as input and returns a new color vector, keeping only the green component from the original color vector and setting the other components to zero. This effectively converts the original color to a grayscale image, using only the green channel information.
//Gray = R*0.3 + G*0.59 + B*0.11
const vec3 floatcoefficient = vec3(0.3, 0.59, 0.11);
vec3 GrayByfloat(vec3 color)
{
float w = dot(color, floatcoefficient);
return vec3(w);
}
The GrayByfloat
function utilizes a constant coefficient vector floatcoefficient
to convert the input color vector color
into a grayscale image. It employs a standard grayscale conversion formula with different weights for the red, green, and blue channels.
//Average
vec3 GrayByAverage(vec3 color)
{
float w = (color.r + color.g + color.b) / 3.0;
return vec3(w);
}
The GrayByAverage
function calculates the average value of the input color vector color
, effectively converting it into a grayscale image.
void mainImage(out vec4 fragColor, in vec2 fragCoord)
{
vec2 uv = fragCoord / iResolution.xy;
vec3 color = texture(iChannel0, uv).rgb;
uv *= vec2(3.0, 1.0);
if (uv.x < 0.95)
{
color = texture(iChannel0, uv).rgb;
color = GrayByGreen(color);
}
else if (uv.x > 1.0 && uv.x < 1.95)
{
uv.x -= 1.0;
color = texture(iChannel0, uv).rgb;
color = GrayByfloat(color);
}
else if (uv.x > 2.0 && uv.x < 3.0)
{
uv.x -= 2.0;
color = texture(iChannel0, uv).rgb;
color = GrayByAverage(color);
}
else
{
color = vec3(1.0);
}
fragColor = vec4(color, 1.0);
}
The mainImage
function is the main function in ShaderToy. It calculates the texture coordinates uv
based on the screen coordinates fragCoord
and retrieves the color from the input texture. Depending on the value of uv.x
, different grayscale conversion methods are applied, and the resulting color is assigned to fragColor
for display on the screen.
「真诚赞赏,手留余香」
真诚赞赏,手留余香
使用微信扫描二维码完成支付
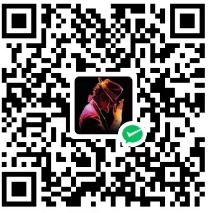