添加鼠标点击事件
绘制位置,pointsize为60
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="glMatrix-0.9.6.min.js"></script>
<script>
class WebGLApp {
constructor() {
this.vertexString = `
attribute vec3 a_position;
uniform mat4 proj;
void main(void){
gl_Position = vec4(a_position, 1.0);
gl_PointSize = 60.0;
}
`;
this.fragmentString = `
precision mediump float;
void main(void){
gl_FragColor = vec4(0.0, 0.0, 1.0, 1.0);
}
`;
this.projMat4 = mat4.create();
this.webgl = null;
this.points = [];
}
init() {
this.initWebgl();
this.initShader();
this.initBuffer();
this.draw();
}
initWebgl() {
let webglDiv = document.getElementById('myCanvas');
this.webgl = webglDiv.getContext("webgl");
this.webgl.viewport(0, 0, webglDiv.clientWidth, webglDiv.clientHeight);
mat4.ortho(0, webglDiv.clientWidth, webglDiv.clientHeight, 0, -1.0, 1.0, this.projMat4);
}
initShader() {
let vsshader = this.webgl.createShader(this.webgl.VERTEX_SHADER);
let fsshader = this.webgl.createShader(this.webgl.FRAGMENT_SHADER);
this.webgl.shaderSource(vsshader, this.vertexString);
this.webgl.shaderSource(fsshader, this.fragmentString);
this.webgl.compileShader(vsshader);
this.webgl.compileShader(fsshader);
if (!this.webgl.getShaderParameter(vsshader, this.webgl.COMPILE_STATUS)) {
var err = this.webgl.getShaderInfoLog(vsshader);
alert(err);
return;
}
if (!this.webgl.getShaderParameter(fsshader, this.webgl.COMPILE_STATUS)) {
var err = this.webgl.getShaderInfoLog(fsshader);
alert(err);
return;
}
let program = this.webgl.createProgram();
this.webgl.attachShader(program, vsshader);
this.webgl.attachShader(program, fsshader);
this.webgl.linkProgram(program);
this.webgl.useProgram(program);
this.webgl.program = program;
}
initBuffer() {
let aPosition = this.webgl.getAttribLocation(this.webgl.program, "a_position");
//监听鼠标点击事件,动态添加点
document.addEventListener("mousedown", (e) => {
let x = e.clientX;
let y = e.clientY;
let rect = e.target.getBoundingClientRect();
let pointx = ((x - rect.left) - 512) / 512;
let pointy = (350 - (y - rect.top)) / 350;
this.points.push(pointx);
this.points.push(pointy);
this.points.push(0);
let pointPosition = new Float32Array(this.points);
let pointBuffer = this.webgl.createBuffer();
this.webgl.bindBuffer(this.webgl.ARRAY_BUFFER, pointBuffer);
this.webgl.bufferData(this.webgl.ARRAY_BUFFER, pointPosition, this.webgl.STATIC_DRAW);
this.webgl.enableVertexAttribArray(aPosition);
this.webgl.vertexAttribPointer(aPosition, 3, this.webgl.FLOAT, false, 0, 0);
this.webgl.clearColor(0.0, 0.0, 0.0, 1.0);
this.webgl.clear(this.webgl.COLOR_BUFFER_BIT | this.webgl.DEPTH_BUFFER_BIT);
this.webgl.drawArrays(this.webgl.POINTS, 0, this.points.length / 3);
});
let uniformProj = this.webgl.getUniformLocation(this.webgl.program, "proj");
this.webgl.uniformMatrix4fv(uniformProj, false, this.projMat4);
}
draw() {
this.webgl.clearColor(0.0, 0.0, 0.0, 1.0);
this.webgl.clear(this.webgl.COLOR_BUFFER_BIT | this.webgl.DEPTH_BUFFER_BIT);
}
}
//这是一个事件处理程序,当整个页面(包括所有依赖资源如图像、脚本等)加载完成后触发。
window.onload = () => {
const app = new WebGLApp();
app.init();
};
</script>
</head>
<body>
<canvas id='myCanvas' width="1024" height='700'></canvas>
</body>
</html>
「真诚赞赏,手留余香」
真诚赞赏,手留余香
使用微信扫描二维码完成支付
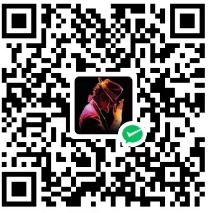